What is Laravel and its use
Categories: Laravel
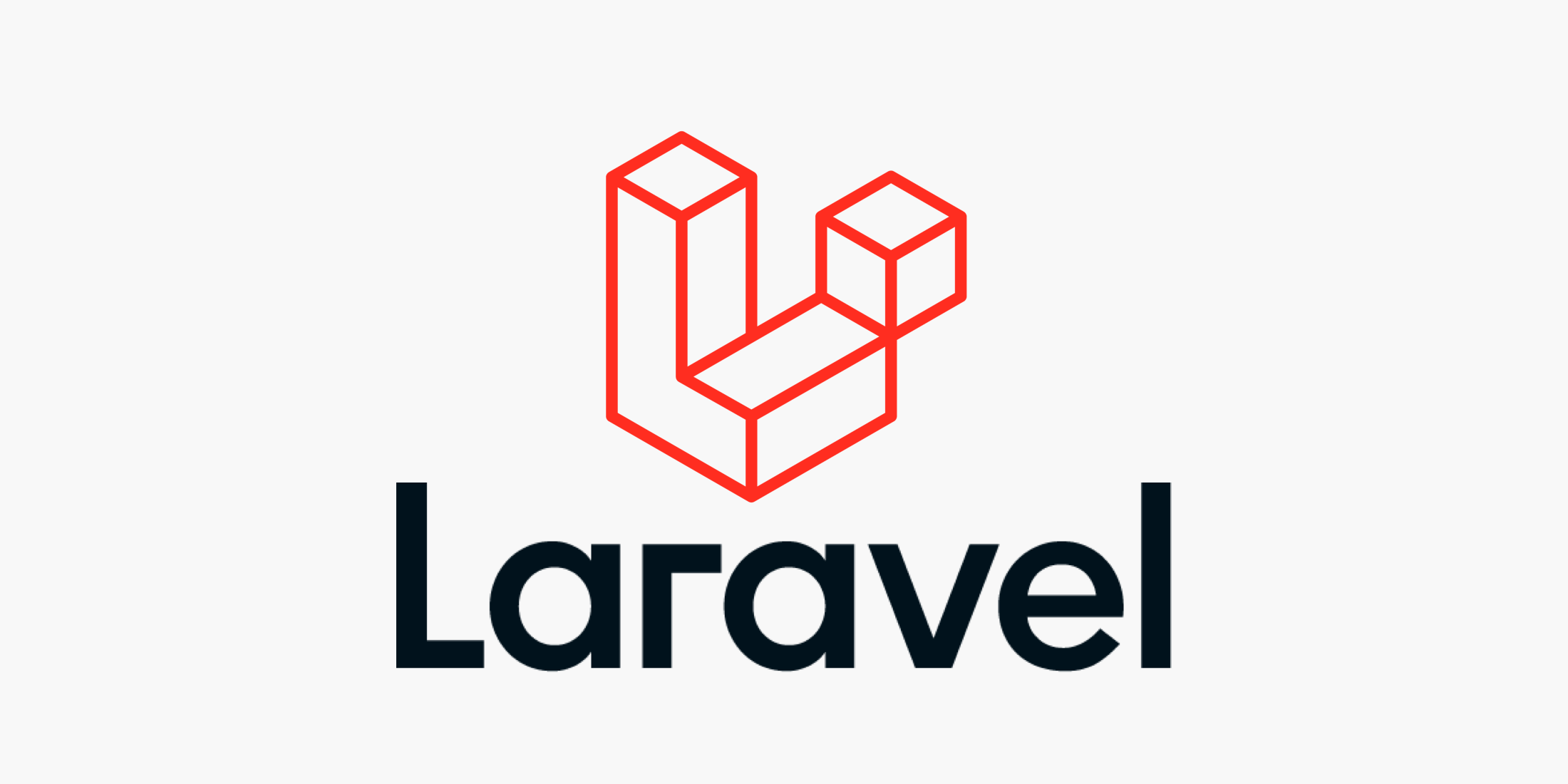
What Is Laravel, And How Do You Get Started with It?
Laravel is a web framework for making custom applications. It runs on PHP, and is entirely free and open source. We’ll discuss what makes this framework a good choice, and why you might want to base your app on it.
What Is Laravel Used for?
Laravel is primarily used for building custom web apps using PHP. It’s a web framework that handles many things that are annoying to build yourself, such as routing, templating HTML, and authentication.
Laravel is entirely server-side, due to running on PHP, and focuses heavily on data manipulation and sticking to a Model-View-Controller design. A framework like React might put most of its attention on user interaction and shiny features, but Laravel simply presents a solid foundation for you to build off of—and does it right.
Laravel is one of the best PHP web frameworks, but there are many other frameworks in different languages. Rails is another server-side rendered framework, similar to Laravel, but based on Ruby. React, Vue, and Angular are all client-side JavaScript frameworks but can be configured to render server-side as well.
Alternatively, if you application leans towards a blog style with multiple text-based posts, you could base it off of WordPress, which also runs on PHP. But Laravel doesn’t force you to use features you don’t want, it just gives you the tools to build something like WordPress by yourself.
How Does Laravel Work?
Laravel uses a design pattern called Model-View-Controller, or MVC.
The “Model” represents the shape of the data your application operates on. If you have a table of users, each with a list of posts they’ve made, that’s your model.
The “Controller” interacts with this model. If a user requests to see their posts page, the controller talks to the model (often just the database) and retrieves the info. If the user wants to make a new post, the controller updates the model. The controller contains most of the logic for your application.
The controller uses that info to construct a “View.” The view is a template with which the model can be plugged into and displayed, and it can be manipulated by the controller. The view is all your application’s HTML components.
Laravel uses this structure to power custom apps. It uses the Blade templating engine, which allows HTML to be broken into pieces and managed by the controller. It all starts with routes, defined in routes/web.php, that handle HTTP requests based on the location being requested. For example, the following function would run if a user requested https://yoursite.com/greeting:
Route::get('/greeting', function () {
return view('greeting', ['name' => 'James']);
});
This route executes a function that returns a view from resources/views/. The view has been passed data (the name variable), which it can use inside the markup:
<!-- View stored in resources/views/greeting.blade.php -->
<html>
<body>
<h1>Hello, {{ $name }}</h1>
</body>
</html>
This is as simple as it gets, but a lot can happen in between the request and the return of a view. Laravel supports middleware, which will run before the request is handled. You could use this to lock down certain pages by checking if a user is authenticated before a request is handled.
Instead of showing a view directly, you can also pass the request off to a controller, which can handle more complex logic before ultimately returning some resource (often a view). You can read more about the inner workings of the Laravel framework in their docs.
How to Get Started
Laravel runs on PHP, which means all you need is a web server like Apache or Nginx with PHP installed. You’ll also need Composer, a dependency manager for PHP, and you’ll need a database. MySQL will work fine, but PostgreSQL and SQLite are supported as well.
Once the dependencies are installed, you can download and install Laravel from Composer:
composer global require laravel/installer
This is technically just the Laravel installer, so you’ll need to create a new Laravel installation using laravel new:
laravel new blog
This creates a new directory named “blog” and installs Laravel to it. This contains a built-in .htaccess file, so all you’ll have to do is make sure mod_rewrite is turned on to enable .htaccess files, and point Apache towards the directory. Alternatively, if you’d just like to get it off the ground, you can use PHP’s built-in Artisan server by running the following command in the project directory:
php artisan serve
This launches a development server at localhost:8000. If it’s running on a server, you’ll have to open that port or use SSH tunnelling to access it. This isn’t a proper web server though, so you’ll still want Apache or Nginx for production.