Previous | Home | Next |
Array
An array is a collection of elements, items or values of variable. It allow to hold more than one value to a single variable. It is a special data type to store the elements.
Creating an Array:
Array is created by using array() function. There are types of array creation:
- Indexed Array
- Associative Array
- Multidimentional Array
It is an array with a numeric index. Values are stored and accessed in indexed array linearly. By default array index starts from zero. There are two ways to represent indexed array as shown in example.
Examle:
<html> <head> <title>indexed array in PHP</title> </head> <body> <?php //First way to represent indexed array. echo "First way to represent indexed array:<br/>"; $days = array("Mon", "Tues", "Wed", "Thurs", "Fri", "Sat", "Sun"); foreach($days as $value) { echo "Day is $value <br />"; } echo "<br/>"; //Second way to represent indexed array. echo "Second way to represent indexed array:<br/>"; $days[0] = "Monday"; $days[1] = "Tuesday"; $days[2] = "Wednesday"; $days[3] = "Thursday"; $days[4] = "Friday"; $days[5] = "Satday"; $days[6] = "Sunday"; foreach( $days as $value ) { echo "Day is $value <br />"; } ?> </body> </html>
Output:
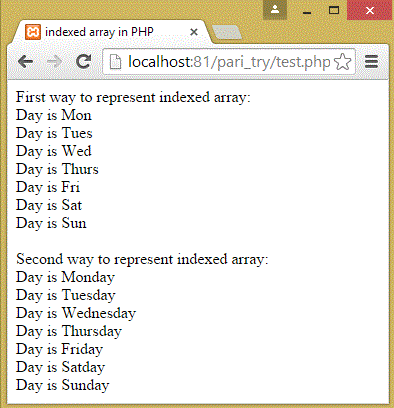
It is an array which uses key. A key is associated with value. There are two ways to represent associative array as shown in example.
Example:
<html> <head> <title>associative array in PHP</title> </head> <body> <?php //First way to represent associative array. echo "First way to represent associative array:<br/>"; $days_week = array ("Mon"=>1,"Tues"=>2,"Wed"=>3,"Thurs"=>4,"Fri"=>5,"Sat"=>6,"Sun"=>7); echo "Day " .$days_week['Mon']." is Monday<br/>"; echo "Day " .$days_week['Tues']." is Tuesday<br/>"; echo "Day " .$days_week['Wed']." is Wednesday<br/>"; echo "Day " .$days_week['Thurs']." is Thursday<br/>"; echo "Day " .$days_week['Fri']." is Friday<br/>"; echo "Day " .$days_week['Sat']." is Saturday<br/>"; echo "Day " .$days_week['Sun']." is Sunday<br/>"; echo "<br/>"; //Second way to represent associative array. echo "Second way to represent indexed array:<br/>"; $days_week["Mon"] = 1; $days_week["Tues"] = 2; $days_week["Wed"] = 3; $days_week["Thurs"] = 4; $days_week["Fri"] = 5; $days_week["Sat"] = 6; $days_week["Sun"] = 7; echo "Day " .$days_week['Mon']." is Monday<br/>"; echo "Day " .$days_week['Tues']." is Tuesday<br/>"; echo "Day " .$days_week['Wed']." is Wednesday<br/>"; echo "Day " .$days_week['Thurs']." is Thursday<br/>"; echo "Day " .$days_week['Fri']." is Friday<br/>"; echo "Day " .$days_week['Sat']." is Saturday<br/>"; echo "Day " .$days_week['Sun']." is Sunday<br/>"; ?> </body> </html>
Output:
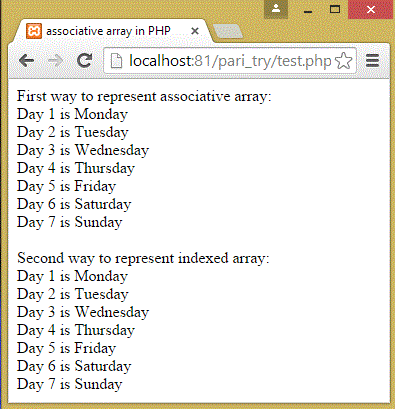
It is an array which holds at least one array as a value. Values in the multi-dimensional array are accessed using multiple index.
Example:
<html> <head> <title>multidimensional array in PHP</title> </head> <body> <?php $marks = array( "Ankur" => array ( "MCA Iyr" => 790, "MCA IIyr" => 850, "MCA IIIyr" => 825 ), "Arsh" => array ( "MCA Iyr" => 765, "MCA IIyr" => 810, "MCA IIIyr" => 799 ), "Deepak" => array ( "MCA Iyr" => 786, "MCA IIyr" => 835, "MCA IIIyr" => 830 ) ); /* Accessing multi-dimensional array values */ echo "Marks for Ankur in MCA IIyear : " ; echo $marks['Ankur']['MCA IIyr'] . "<br />"; echo "Marks for Arsh in MCA Iyear : "; echo $marks['Arsh']['MCA Iyr'] . "<br />"; echo "Marks for Deepak in MCA IIIyear : " ; echo $marks['Deepak']['MCA IIIyr'] . "<br />"; ?> </body> </html>
Output:
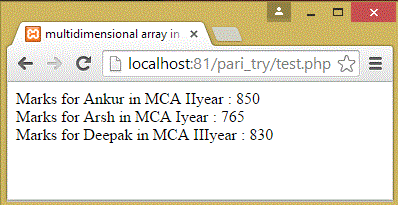
Previous | Home | Next |