Previous | Home | Next |
How to write a first C program
Rules:
- In C program each instruction written as a separate statement.
- The statement of a program must be in the sequence by which we want to execute.
- Blank space are not allowed in the variable name but you can use blank spaces between two words which increase readability.
- Statements should be in small case letters.
- Every statement ends with a semicolon (;).
- Semicolon is called statement terminator.
- You can put the comment within a program by using /* …….*/.
- Comments are not necessary to use in a program but it is a good habit to use comments in a program.
Compilation and Execution of a C Program:
- Write a program using editor.
- Save the file with .c extension.
- To compile the program you can select compile from compile menu and press enter or you can press Alt+f9
- It will check the errors if program has any error it will terminate the program then you can find out he error and you can correct it. After correcting the error you can compile the program again. After compiling successfully it will create a object (.obj) file.
- Once the source program has been converted into an object file, it is still not in the form that you can run. The reason behind this is that there may be some references to standard library functions or user-defined functions in other object files, which are compiled separately. Therefore these object files are to be linked together along with standard library functions that are contained in library files. To link our object file, select Link from the Compile menu. After this the .OBJ file will be compiled with the one or more library files. The result of this linking process produces an executable file with .EXE extension. Figure-4 depicts this linking process.
- .exe file is a stand alone executable file. which can direct execute from command prompt
- After pressing Alt+f9 it will complete the whole process creating .OBJ and .EXE.
- To display the output of the program press Alt+f5.
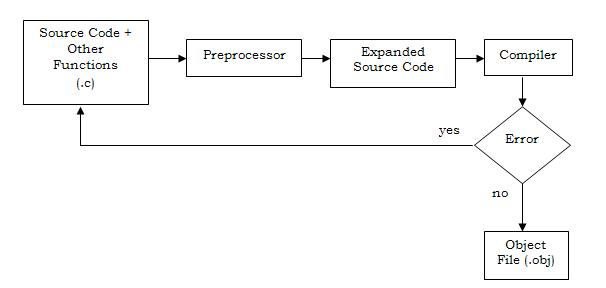
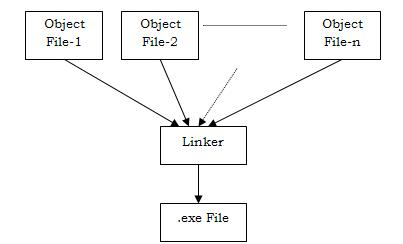
Examples of a simple C program:
/* Calculation of simple interest */ main( ) { int p, n ; float r, si ; p = 1000 ; n = 3 ; r = 8.5 ; /* formula for simple interest */ si = p * n * r / 100 ; printf ( "%f" , si ) ; }
main():
- main() is a function which is entry point of any program.
- main() is collective name which is given to a set of statements.
- This name has to be main(). It can not be change.
- Statements within a main() should be enclosed within a pair of { }.
- Every function used in a program must be declared.
main() { statement 1; statement 2; }
Difference between main() and other functions:
- parameters to main() are passed from command line,
- main() is the only function declared by the compiler and defined by the user,
- main() is by convention a unique external function,
- main() is the only function with implicit return 0; at the end of main(). When control crosses the ending ‘}’ for main it returns control to the operating system by returning 0 to it (if there is no explicit return statement with a return value). The OS normally treats return 0 as the successful termination of the program.
- return type for main() is always is an int, (some compilers may accept void main() or any other return type, but they are actually treated as if it is declared as int main(). It makes the code non-portable. Always use int main().
Example:
main() { Int i; Static int j; }
NOTES:
- Parameters are passed from command line to main ().
- It is the only function declares by the compiler and defines by the user.
- Return type for main() is always is an int, (some compilers may accept void main() or any other return type, but they are actually treated as if it is declared as int main(). It makes the code non-portable. Always use int main() ).
Receiving Input:
To receive the input from user we use scanf() function in C Language. scanf() is a function that reads data with specified format and is present in many other programming languages.
int scanf(const char *format, ...);"scanf" stands for "scan format", because it scans the input for valid tokens and parses them according to a specified format.
#include <stdio.h> int main(void) { int n; while (scanf("%d", &n) == 1) printf("%d\n", n); return 0; }
Previous | Home | Next |