Previous | Home | Next |
Forms Validate in PHP
The PHP filters provide an easy way to sanitize and validate the form data. Proper validation of form data is important to protect your form from hackers and spammers.
Text Fields and Text Areas
Any fields are text input elements are known as text fields and text areas.
Example:
Name: <input type="text" name="name"><br /> <br /> E-mail: <input type="text" name="email"><br /> <br /> skills: <textarea name="Skills" rows="5" cols="40"></textarea>
Radio Button
Radio Button allows user to choose one option from the given choices.
Example:
Choose Colour:<br /> <input type="radio" name="colour" value="red">Red<br /> <input type="radio" name="colour" value="blue">Blue<br /> <input type="radio" name="colour" value="pink">Pink<br /> <input type="radio" name="colour" value="orange">Orange<br /> <input type="radio" name="colour" value="green">Green<br /> <input type="radio" name="colour" value="purple">Purple<br /> <input type="radio" name="colour" value="black">Black<br /> <input type="radio" name="colour" value="white">White<br />
<html> <head> <title>PHP FORM</title> </head> <body> <?php // define variables and set to empty values $name = $email = $gender = $contact = ""; if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = test_input($_POST["name"]); $email = test_input($_POST["email"]); $contact = test_input($_POST["contact"]); $gender = test_input($_POST["gender"]); } function test_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } ?> <h2>Information Form:</h2> <form method="post" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>"> Name: <input type="text" name="name"> <br><br> E-mail: <input type="text" name="email"> <br><br> Contact: <input type="text" name="contact"> <br><br> Gender: <input type="radio" name="gender" value="female">Female <input type="radio" name="gender" value="male">Male <br><br> <input type="submit" name="submit" value="Submit"> </form> <?php echo "<h2>Your Information is:</h2>"; echo $name; echo "<br>"; echo $email; echo "<br>"; echo $contact; echo "<br>"; echo $gender; ?> </body> </html>
Output:
Before Submit Information:
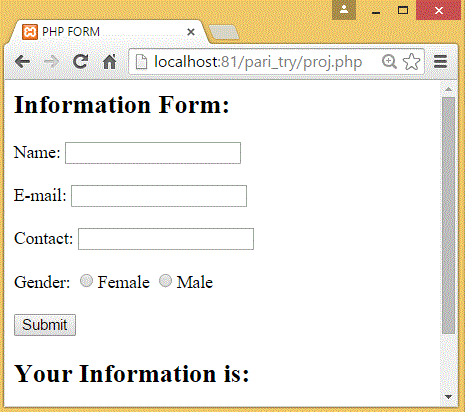
After Submit Information:
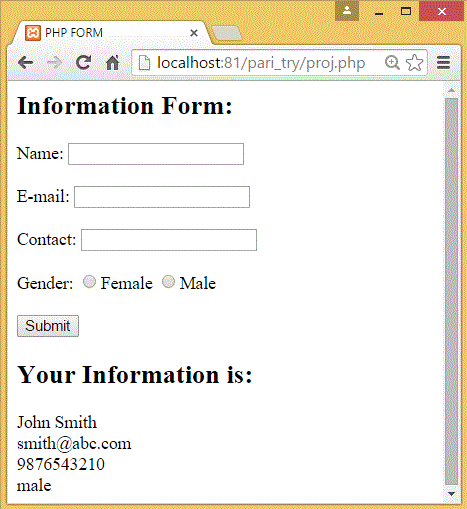
Previous | Home | Next |