Notepad Layout Using Java Frame
Here we are going to
create the layout of notepad using java frame. In this example we are creating
Layout only. The working will in other example .We are simply creating menu bar
and adding menu items .We are using window listener to handle the window events.
To make MenuBar and add MenuItems & Menu we are using
following steps:
Create object of MenuBar:
To create menu bar we have MenuBar class.
Syntax to create MenuBar:
MenuBar mb=new MenuBar();
Now we have to set
the menu bar .To set menu bar we have setMenuBar( MenuBar o)
method.
Syntax to set
MenuBar:
setMenuBar(mb);
In this example we
are adding menubar as setMenuBar(mb). To create menu we have a
Menu class. In this example we are creating three menu "File","Edit"
and "Help".
Syntax to create Menu:
Menu f1=new Menu("File");
To add items into these menu we need to create object of MenuItem and to
add these menu items we have add() method.
Syntax to create MenuItem:
MenuItem a1=new MenuItem("N e w");
To add MenuItem:
f1.add(a1);
Here we are using
addSeparator() method to separate the menu items.
Here we are using TextArea class to make text area.
We implements class by WindowListener interface. As we know
this listener have seven methods which we must have overwrite.
public void
windowOpened(WindowEvent e):
This methods automatically called when window is opened.
public void
windowClosing(WindowEvent e)
This method is automatically called when window is going to closed.
public void
windowClosed(WindowEvent e)
This method is automatically called when window is finally closed.
public void
windowActivated(WindowEvent e)
This method is automatically called when window is activated.
public void
windowDeactivated(WindowEvent e)
This method is called when window is deactivated
public void
windowIconified(WindowEvent e)
This method is called when window is minimums
public void
windowDeiconified(WindowEvent e)
This method is automatically called when method is maximums
We are using addWindowListener(this) method to register the
window listener.
Here we are using hide() method to hide the window's .This methods is called when
click on closed button. As we know in applet the closed button is not work on
clicked by mouse .So we have handle it by window event . We are calling hide()
to hide the window on click.
SOURCE CODE
import java.awt.*;
import java.awt.event.*;
public class NotepadLayout extends Frame implements WindowListener
{
MenuBar mb = new MenuBar();
Menu f1 = new Menu("File");
Menu f2 = new Menu("Edit");
Menu f3 = new Menu("Help");
MenuItem a1 = new MenuItem("N e w");
MenuItem a2 = new MenuItem("O p e n");
MenuItem a3 = new MenuItem("S a v e");
MenuItem a4 = new MenuItem("E x i t");
MenuItem a5 = new MenuItem("C u t");
MenuItem a6 = new MenuItem("C o p y ");
MenuItem a7 = new MenuItem("P a s t e ");
MenuItem a8= new MenuItem("S e l e c t A l l ");
MenuItem a9 = new MenuItem("H e l p T o p i c");
TextArea ta = new TextArea();
NotepadLayout()
{
setTitle("Notepad developed by ............");
f1.add(a1);
f1.addSeparator();
f1.add(a2);
f1.addSeparator();
f1.add(a3);
f1.addSeparator();
f1.add(a4);
f2.add(a5);
f2.addSeparator();
f2.add(a6);
f2.addSeparator();
f2.add(a7);
f2.addSeparator();
f2.add(a8);
f3.add(a9);
mb.add(f1);
mb.add(f2);
mb.add(f3);
setMenuBar(mb);
ta.setFont(new Font("Courier New",1,16));
add(ta);
setSize(400,300);
addWindowListener(this);
show();
}
public void windowOpened(WindowEvent e)
{
System.out.println("Window Opened");
}
public void windowClosing(WindowEvent e)
{
System.out.println("Window Closing");
hide();
}
public void windowClosed(WindowEvent e)
{
System.out.println("Window Closed");
}
public void windowActivated(WindowEvent e)
{
System.out.println("Window Activated");
}
public void windowDeactivated(WindowEvent e)
{
System.out.println("Window Dectivated");
}
public void windowIconified(WindowEvent e)
{
System.out.println("Window Minimize");
}
public void windowDeiconified(WindowEvent e)
{
System.out.println("Window Maximize");
}
public static void main(String aa[])
{
NotepadLayout n = new NotepadLayout();
}
}
|
Download Source File
Output of This Example
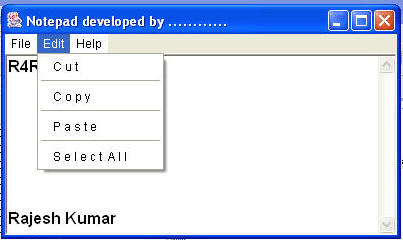