Previous | Home | Next |
JavaScript provide Error handling that is the process of catching the errors generated by our program It can be used to handle all or some of the errors that can occur in a script.
There are manely three types of errors in javascript
- Syntax Errors
- Runtime Errors
- Logical Errors
There are several ways to test and find errors in Javascript
- The Try.....Catch statement
- The throw keyword
- The onerror event handler
The try block Contains code to be executed.
The catch block Contains code to be executed if an error occurs in the code within the try block.
The throw block allows you to create a custom error.
The onerror event its handle the first feature to facilitate error handling
Syntax of try catch :
try{ code to be executed } catch (error){ code to be executed if an error occurs in the code within the try block }
Example :
<html> <head> <script > function myFunc() { var a = 500; try { alert("Value of variable a is : " + a ); } catch ( c ) { alert("Error: " + c.description ); } } </script> </head> <body> <p>Click the following to see the result:</p> <form> <input type="button" value="Click " onclick="myFunc();" /> </form> </body> </html>
Output :
Click the following to see the result:
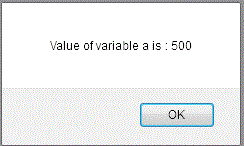
Example of onerror event
<html> <head> <script> window.onerror = function () { alert("An error occurred."); } </script> <body> <p>Click the following to see the result:</p> <form> <input type="button" value="Click " onclick="myFunc();" /> </form> </head> </body> </html>
Output :
Click the following to see the result:
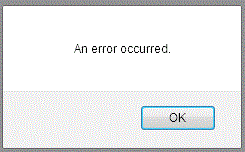
Previous | Home | Next |